ESP32 可以當做 Wi-Fi station、AP或兩者兼而有之。在本課程中,將向您展示如何使用 Arduino IDE 將 ESP32 設置為AP。

多數使用 ESP32 的項目中,我們都將 ESP32 連接到本地端的無線路由器。這樣我們就可以通過本地的網路訪問 ESP32。
在這種情況下,路由器做為網路的AP(接入點),ESP32 設置為station(站點)。在這種情況下,您需要連接到路由器(本地網路)來控制 ESP32。

但是,如果您將 ESP32 設置為AP(接入點),這樣您就可以使用任何具有 Wi-Fi 功能的設備連接到 ESP32,而無需連接到路由器。
簡單講,當您將 ESP32 設置為 AP 時,您就會建立自己的 Wi-Fi 網路,而附近的 Wi-Fi 設備(站)可以連接到它(例如您的智能手機或計算機)。
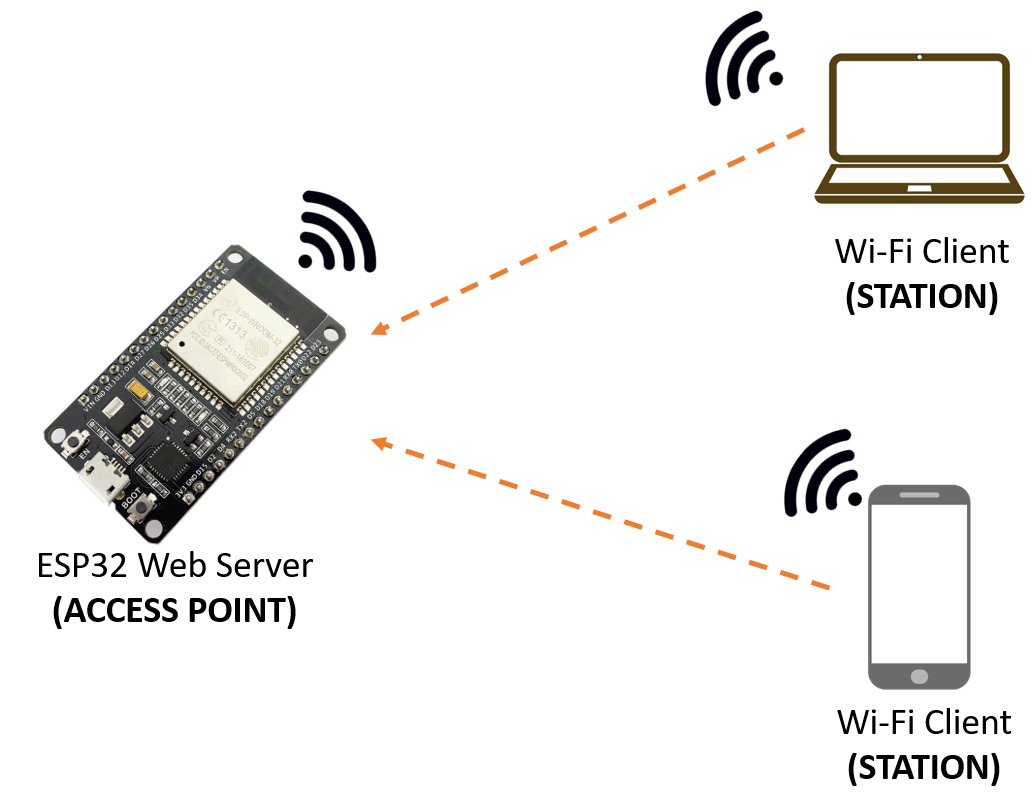
在此,我們將向您展示如何將 ESP32 設置為 Web 服務器項目中的AP(接入點)。這樣,您無需連接到路由器即可控制您的 ESP32。因為 ESP32 沒有進一步連接到有線網路(如路由器),所以它被稱為softAP。
內容目錄
ToggleESP32 AP
在此範例中,我們將新增AP功能。我們將在此處向您展示的內容可用於任何 ESP32 Web 服務器範例。
上傳下面提供的程式碼以將 ESP32 設置為AP。
/*********
Rui Santos
Complete project details at https://randomnerdtutorials.com
*********/
// Load Wi-Fi library
#include <WiFi.h>
// Replace with your network credentials
const char* ssid = "ESP32-Access-Point";
const char* password = "123456789";
// Set web server port number to 80
WiFiServer server(80);
// Variable to store the HTTP request
String header;
// Auxiliar variables to store the current output state
String output26State = "off";
String output27State = "off";
// Assign output variables to GPIO pins
const int output26 = 26;
const int output27 = 27;
void setup() {
Serial.begin(115200);
// Initialize the output variables as outputs
pinMode(output26, OUTPUT);
pinMode(output27, OUTPUT);
// Set outputs to LOW
digitalWrite(output26, LOW);
digitalWrite(output27, LOW);
// Connect to Wi-Fi network with SSID and password
Serial.print("Setting AP (Access Point)…");
// Remove the password parameter, if you want the AP (Access Point) to be open
WiFi.softAP(ssid, password);
IPAddress IP = WiFi.softAPIP();
Serial.print("AP IP address: ");
Serial.println(IP);
server.begin();
}
void loop(){
WiFiClient client = server.available(); // Listen for incoming clients
if (client) { // If a new client connects,
Serial.println("New Client."); // print a message out in the serial port
String currentLine = ""; // make a String to hold incoming data from the client
while (client.connected()) { // loop while the client's connected
if (client.available()) { // if there's bytes to read from the client,
char c = client.read(); // read a byte, then
Serial.write(c); // print it out the serial monitor
header += c;
if (c == '\n') { // if the byte is a newline character
// if the current line is blank, you got two newline characters in a row.
// that's the end of the client HTTP request, so send a response:
if (currentLine.length() == 0) {
// HTTP headers always start with a response code (e.g. HTTP/1.1 200 OK)
// and a content-type so the client knows what's coming, then a blank line:
client.println("HTTP/1.1 200 OK");
client.println("Content-type:text/html");
client.println("Connection: close");
client.println();
// turns the GPIOs on and off
if (header.indexOf("GET /26/on") >= 0) {
Serial.println("GPIO 26 on");
output26State = "on";
digitalWrite(output26, HIGH);
} else if (header.indexOf("GET /26/off") >= 0) {
Serial.println("GPIO 26 off");
output26State = "off";
digitalWrite(output26, LOW);
} else if (header.indexOf("GET /27/on") >= 0) {
Serial.println("GPIO 27 on");
output27State = "on";
digitalWrite(output27, HIGH);
} else if (header.indexOf("GET /27/off") >= 0) {
Serial.println("GPIO 27 off");
output27State = "off";
digitalWrite(output27, LOW);
}
// Display the HTML web page
client.println("<!DOCTYPE html><html>");
client.println("<head><meta name=\"viewport\" content=\"width=device-width, initial-scale=1\">");
client.println("<link rel=\"icon\" href=\"data:,\">");
// CSS to style the on/off buttons
// Feel free to change the background-color and font-size attributes to fit your preferences
client.println("<style>html { font-family: Helvetica; display: inline-block; margin: 0px auto; text-align: center;}");
client.println(".button { background-color: #4CAF50; border: none; color: white; padding: 16px 40px;");
client.println("text-decoration: none; font-size: 30px; margin: 2px; cursor: pointer;}");
client.println(".button2 {background-color: #555555;}</style></head>");
// Web Page Heading
client.println("<body><h1>ESP32 Web Server</h1>");
// Display current state, and ON/OFF buttons for GPIO 26
client.println("<p>GPIO 26 - State " + output26State + "</p>");
// If the output26State is off, it displays the ON button
if (output26State=="off") {
client.println("<p><a href=\"/26/on\"><button class=\"button\">ON</button></a></p>");
} else {
client.println("<p><a href=\"/26/off\"><button class=\"button button2\">OFF</button></a></p>");
}
// Display current state, and ON/OFF buttons for GPIO 27
client.println("<p>GPIO 27 - State " + output27State + "</p>");
// If the output27State is off, it displays the ON button
if (output27State=="off") {
client.println("<p><a href=\"/27/on\"><button class=\"button\">ON</button></a></p>");
} else {
client.println("<p><a href=\"/27/off\"><button class=\"button button2\">OFF</button></a></p>");
}
client.println("</body></html>");
// The HTTP response ends with another blank line
client.println();
// Break out of the while loop
break;
} else { // if you got a newline, then clear currentLine
currentLine = "";
}
} else if (c != '\r') { // if you got anything else but a carriage return character,
currentLine += c; // add it to the end of the currentLine
}
}
}
// Clear the header variable
header = "";
// Close the connection
client.stop();
Serial.println("Client disconnected.");
Serial.println("");
}
}
自定義 SSID 和密碼
您需要定義 SSID 名稱和密碼才能訪問 ESP32。在本範例中,我們將 ESP32 SSID 名稱設置為ESP32-Access-Point, 但您可以將名稱修改為您想要的任何名稱。密碼是123456789, 您也可以修改它。
// You can customize the SSID name and change the password
const char* ssid = "ESP32-Access-Point";
const char* password = "123456789";
將 ESP32 設置為AP
setup()裡面有一段設置 ESP32 作為AP使用softAP()方法:
WiFi.softAP(ssid, password);
您還可以將其他可選參數傳遞給softAP()方法。這是所有參數:
.softAP(const char* ssid, const char* password, int channel, int ssid_hidden, int max_connection)
- SSID(開始定義):最多 63 個字符;
- 密碼(開始定義):最少 8 個字符;如果您希望訪問點打開,請設置為 NULL
- channel: Wi-Fi 頻道號 (1-13)
- ssid_hidden: (0 = 廣播 SSID, 1 = 隱藏 SSID)
- max_connection:最大同時連接的客戶端(1-4)
接下來,我們需要使用softAPIP()方法並在序列埠監控視窗中列印。
IPAddress IP = WiFi.softAPIP();
Serial.print("AP IP address: ");
Serial.println(IP);
這些是您需要包含在 Web 服務器程式碼中以將 ESP32 設置為AP接入點的程式碼片段。
所需零件
您將需要下列零件:
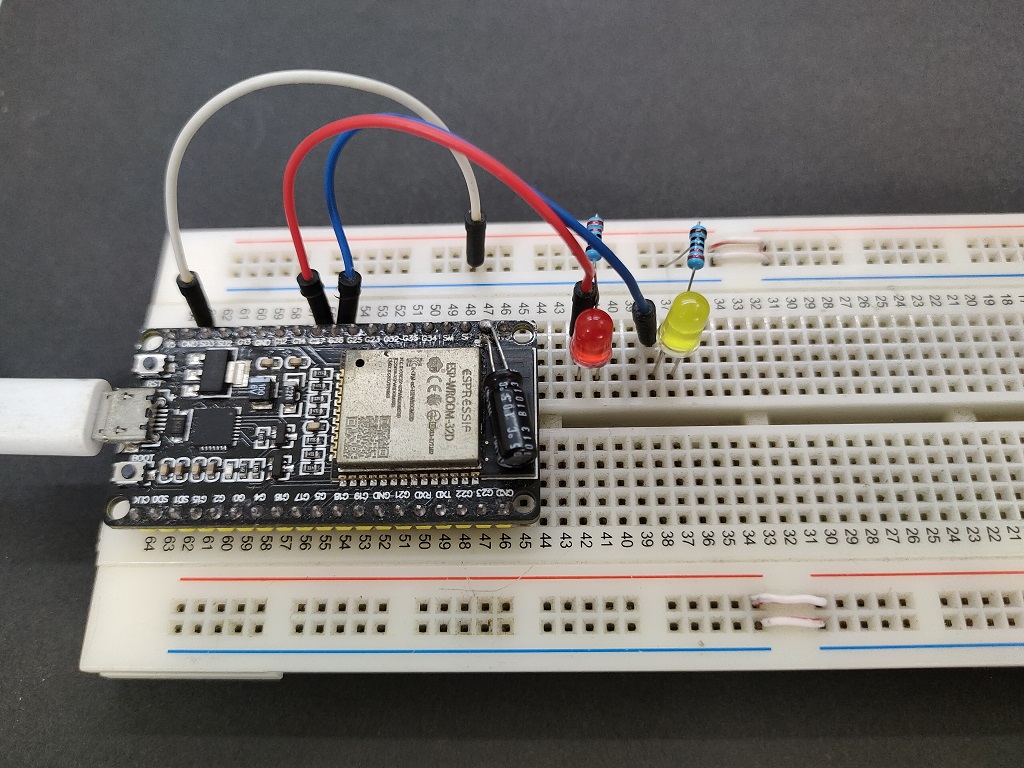
- ESP32 開發板
- 2x 5mm LED
- 2x 330 歐姆電阻
- 麵包板
- 跳線
示意圖
從建構電路開始。將兩個 LED 連接到 ESP32,如下圖所示 – 一個 LED 連接到通用輸入輸出接口 26, 另一個到通用輸入輸出接口 27.
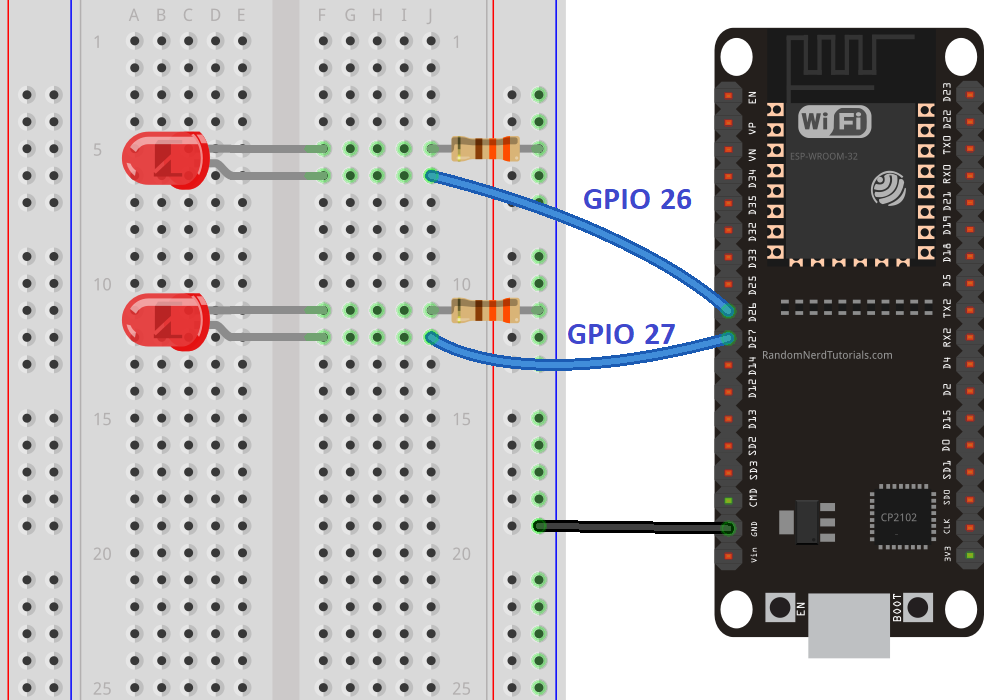
ESP32 IP 地址
將程式碼上傳到您的 ESP32(確保您選擇了正確的開發板和 COM 端口)。以 115200 的鮑率打開序列埠監控視窗。按下 ESP32 的“Enable”按鈕。
您需要訪問 ESP32 AP的 IP 地址將被列印出來。在這種情況下,它是192.168.4.1。
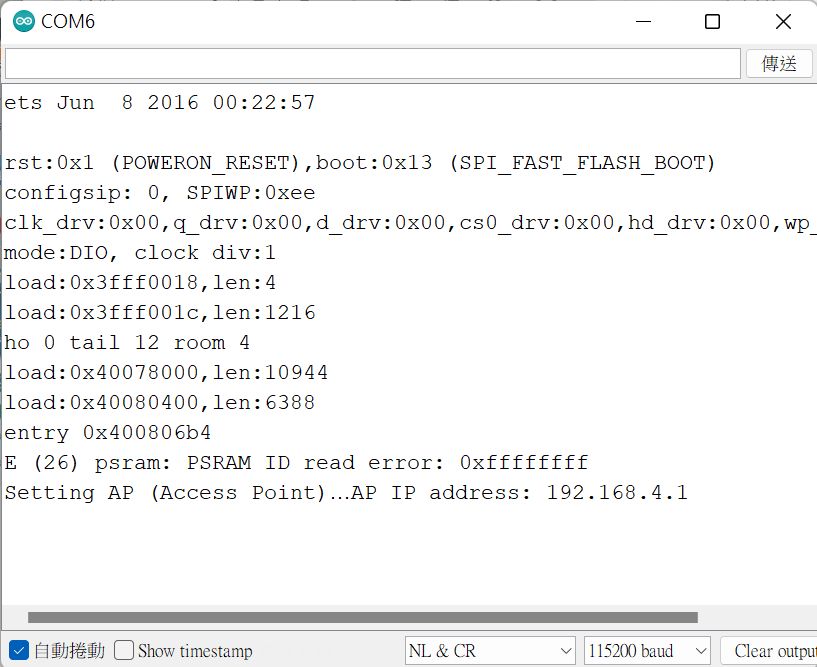
連接到 ESP32 AP
讓 ESP32 運行,在手機中打開 Wi-Fi 設置並點擊 ESP32-Access-Point 網絡:
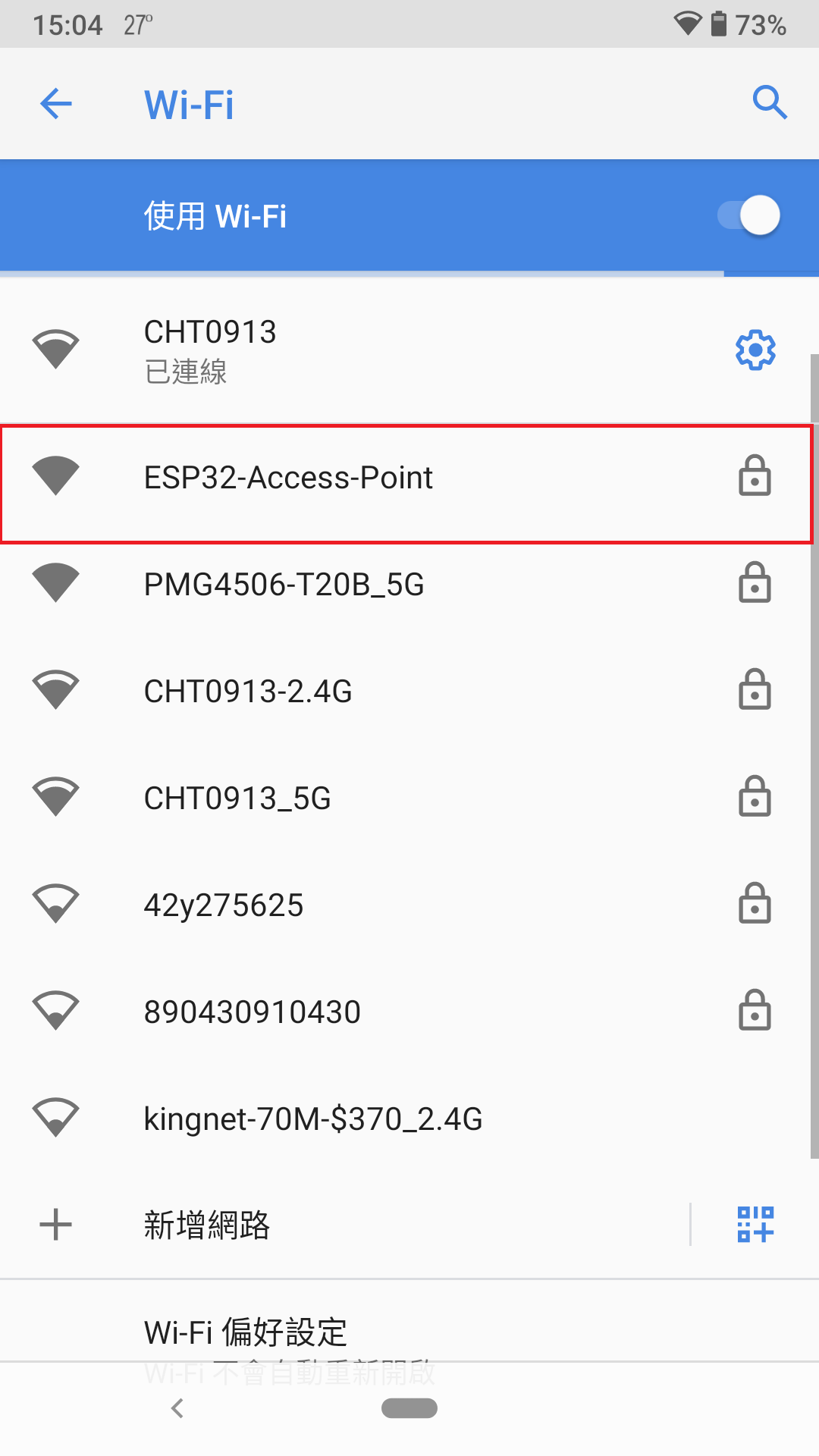
輸入您之前在程式碼中定義的密碼。
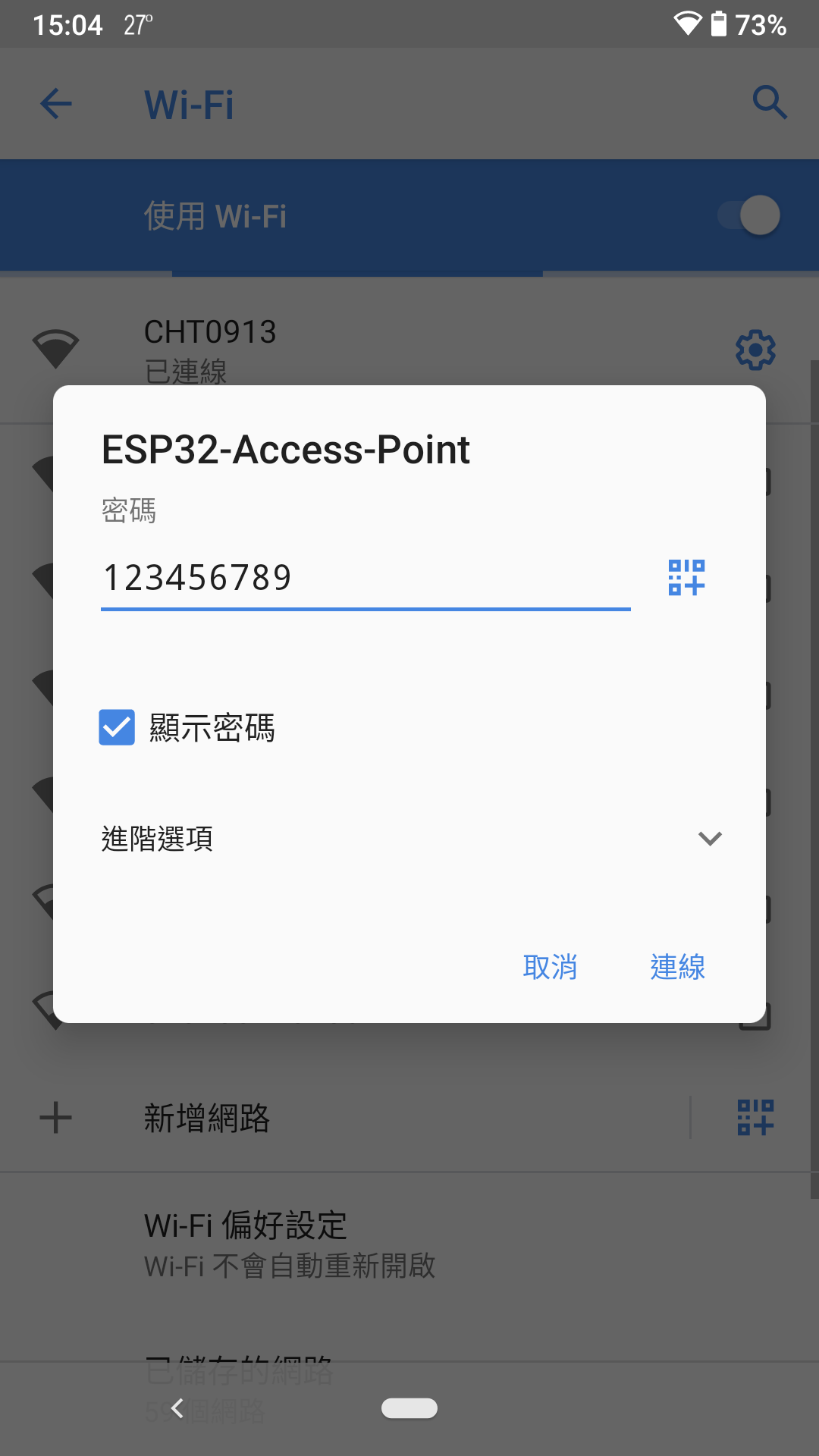
打開您的網路瀏覽器並輸入 IP 地址 192.168.4.1。Web 服務器頁面應加載:
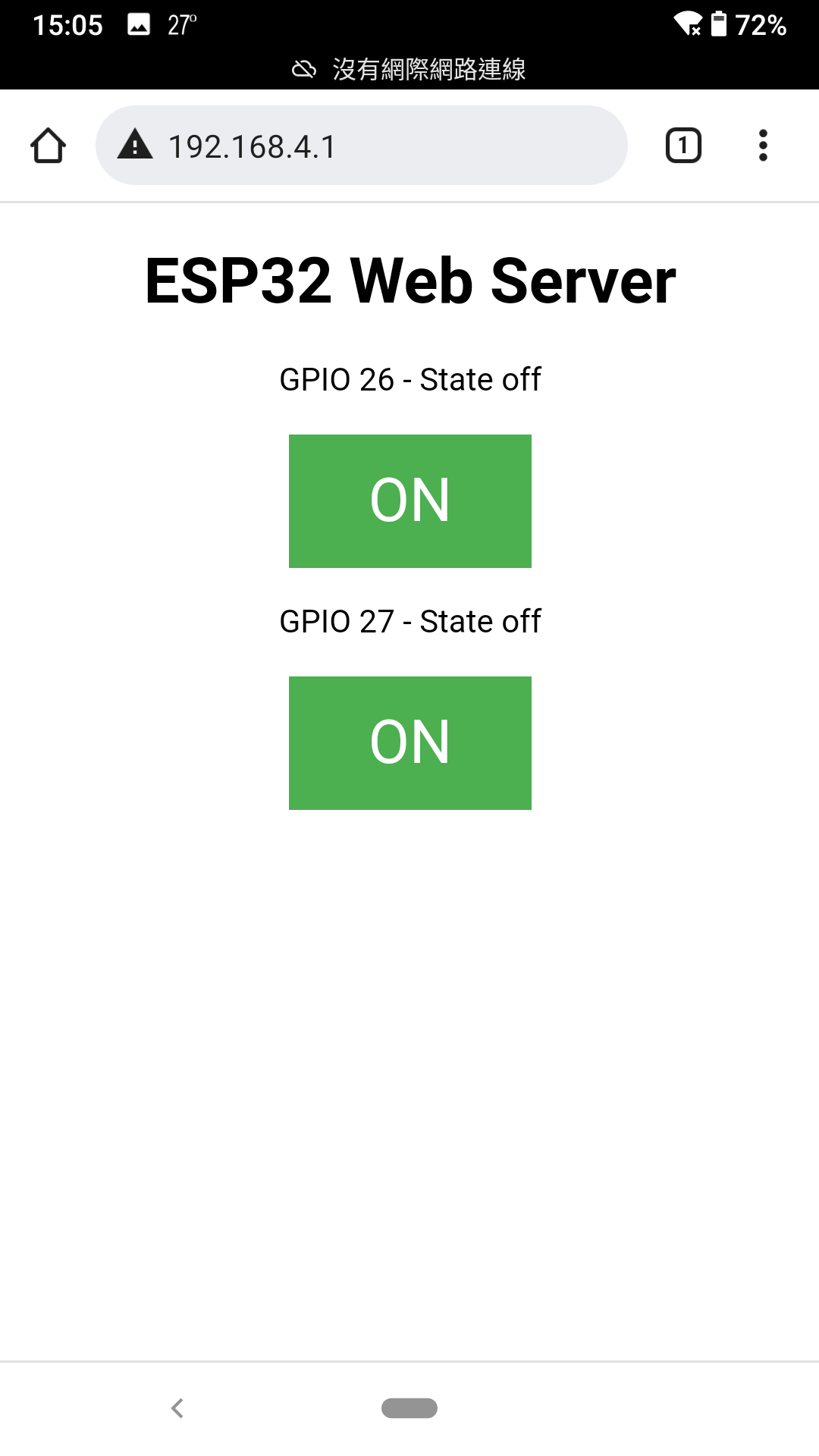
總結
這個簡單的課程向您展示如何將 ESP32 設置為 Web 服務器上的AP。當 ESP32 設置為AP時,具有 Wi-Fi 功能的設備可以直接連接到 ESP32,而無需連接到路由器上。